DERPANET
The Kernel
The kernel, manages the lower part of the network stack, specifically up to layer 3 (UDP/TCP).
Talking to the Kernel with C
When interacting with the kernel using C, you can use these functions to do TCP/UDP socket operations:
1
2
3
4
5
6
|
int socket(int domain, int type, int protocol); //creates UDP or TCP sockets.
int connect(int socket, struct sockaddr *address, int addr_len); // establishes a connection to a TCP socket on a different node.
int send(int socket, char *message, int msg_len, int flags); // sends a message on an opened socket.
int recv(int socket, char *buffer, int buf_len, int flags); //reads data in via the opened socket.
|
For more details and working examples, refer to this
resource.
eBPF
eBPF (Extended Berkeley Packet Filter) provides a safer approach to
building kernel modules, though it can be challenging to work with due
to the absence of a debugger and dynamic memory allocation.
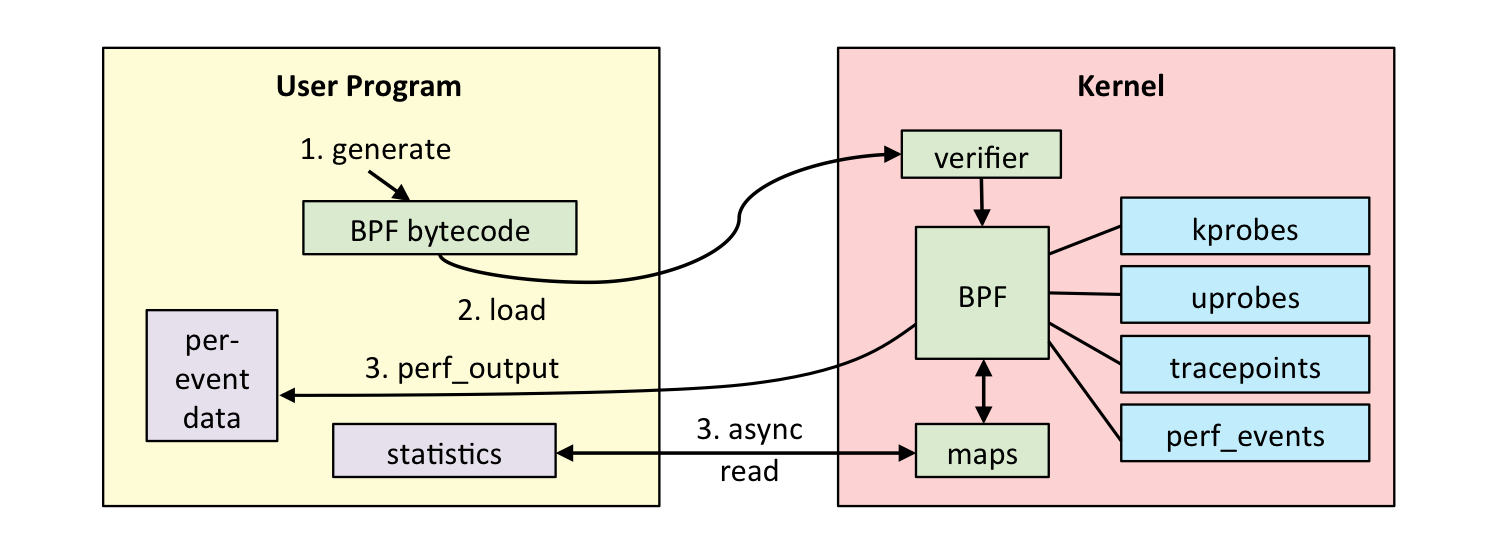
XDP
XDP (eXpress Data Path) is an eBPF thing that allows you to do networking within the
kernel, without having to do Kernal Module stuff.
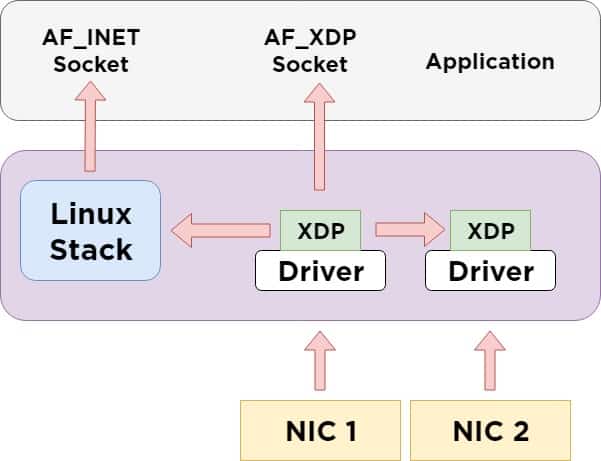
XDP Firewall Example
For a practical example of an XDP firewall, you can explore the source
code here.
What follows are some of my own code that I have simplified.
Kernal Bit
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
|
SEC("xdp_drop")
int xdp_drop_prog(struct xdp_md *ctx) {
// Initialize data.
void *data_end = (void *)(long)ctx->data_end;
void *data = (void *)(long)ctx->data;
int action;
action = parse_l2(data,data_end);
// Scan ethernet header.
struct ethhdr *eth = data;
// Check if the ethernet header is valid.
if (eth + 1 > (struct ethhdr *)data_end) {
return XDP_DROP;
}
char dest_str[20], src_str[20];
build_MAC(eth->h_dest, dest_str);
build_MAC(eth->h_source,src_str);
bpf_printk("Dest: %s", dest_str);
bpf_printk("Source: %s", src_str);
bpf_printk("Proto: %04x", eth->h_proto);
return XDP_PASS;
}
|
Find Out What Is Going On
For network-related inquiries, commands like ip
or ifconfig
(the old
version, not ipconfig
used in Windows) can provide valuable
information.
ip a
- displays the state and addresses of each interface.
Additionally, ip neighbour
reveals the ARP table, essential for
translating MAC addresses to IPv4 addresses.
Debugging
Several tools aid in debugging:
dig
- a DNS debugging tool, e.g., dig @8.8.8.8 compsoc.io
will return:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
; <<>> DiG 9.18.19-1~deb12u1-Debian <<>> @8.8.8.8 compsoc.io
; (1 server found)
;; global options: +cmd
;; Got answer:
;; ->>HEADER<<- opcode: QUERY, status: NOERROR, id: 22746
;; flags: qr rd ra; QUERY: 1, ANSWER: 1, AUTHORITY: 0, ADDITIONAL: 1
;; OPT PSEUDOSECTION:
; EDNS: version: 0, flags:; udp: 512
;; QUESTION SECTION:
;compsoc.io. IN A
;; ANSWER SECTION:
compsoc.io. 300 IN A 76.76.21.21
;; Query time: 24 msec
;; SERVER: 8.8.8.8#53(8.8.8.8) (UDP)
;; WHEN: Mon Nov 13 02:43:47 GMT 2023
;; MSG SIZE rcvd: 55
|
ping
- sends ICMP packets and expects a response
will return:
1
2
3
4
5
6
7
8
9
10
|
PING compsoc.io (76.76.21.21) 56(84) bytes of data.
64 bytes from 76.76.21.21 (76.76.21.21): icmp_seq=1 ttl=239 time=17.1 ms
64 bytes from 76.76.21.21 (76.76.21.21): icmp_seq=2 ttl=239 time=17.6 ms
64 bytes from 76.76.21.21 (76.76.21.21): icmp_seq=3 ttl=239 time=17.0 ms
64 bytes from 76.76.21.21 (76.76.21.21): icmp_seq=4 ttl=239 time=17.5 ms
64 bytes from 76.76.21.21 (76.76.21.21): icmp_seq=5 ttl=239 time=17.8 ms
--- compsoc.io ping statistics ---
5 packets transmitted, 5 received, 0% packet loss, time 4007ms
rtt min/avg/max/mdev = 16.966/17.375/17.777/0.312 ms
|
traceroute
- traces the route of ICMP packets with gradually
increasing TTLs
not going to show you this I don’t want to leak any public IPs ;)
Network-related tasks can be efficiently managed using tools such as
nmtui
, nmcli
, and NetworkManager
, which offer a standard way to
edit networking configurations on Linux without manually editing files
and blowing things up.
NetworkManager
- the package name that contains these tools and a few others
nmtui
- a interactive terminal interface that does all the basics
nmcli
- a more powerfull cli interface for managing network stuff
Userland Config Files
The /etc/hosts
file serves to match up host names to IP addresses,
functioning like a local DNS.
1
2
3
4
5
6
7
8
9
10
11
12
|
127.0.0.1 localhost
127.0.1.1 my-py-hostname
# Kill google!
0.0.0.0 google.com
# The following lines are desirable for IPv6 capable hosts
::1 localhost ip6-localhost ip6-loopback
ff02::1 ip6-allnodes
ff02::2 ip6-allrouters
|
DNS
DNS is often distro spesific. However this is generally done using a config file.
You can find your current config in /etc/resolv.conf
, howver you generally use network manager to do this stuff:
1
2
3
4
|
# Generated by NetworkManager
search home
nameserver 192.168.1.254
nameserver fe80::f6ca:e7ff:fe6e:6edc%wlp3s0
|
Easiest way is just through nmtui
and then editing your connection and manually setting your DNS servers.
Firewalls
iptables
, a fundamental firewall tool, operates with a kernel module
intercepting incoming and outgoing packets, influencing their routing,
and allowing the option to drop packets.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
|
Chain INPUT (policy ACCEPT)
target prot opt source destination
LIBVIRT_INP all -- anywhere anywhere
Chain FORWARD (policy ACCEPT)
target prot opt source destination
DOCKER-USER all -- anywhere anywhere
DOCKER-ISOLATION-STAGE-1 all -- anywhere anywhere
ACCEPT all -- anywhere anywhere ctstate RELATED,ESTABLISHED
DOCKER all -- anywhere anywhere
ACCEPT all -- anywhere anywhere
ACCEPT all -- anywhere anywhere
ACCEPT all -- anywhere anywhere ctstate RELATED,ESTABLISHED
DOCKER all -- anywhere anywhere
ACCEPT all -- anywhere anywhere
ACCEPT all -- anywhere anywhere
ACCEPT all -- anywhere anywhere ctstate RELATED,ESTABLISHED
DOCKER all -- anywhere anywhere
ACCEPT all -- anywhere anywhere
ACCEPT all -- anywhere anywhere
ACCEPT all -- anywhere anywhere ctstate RELATED,ESTABLISHED
DOCKER all -- anywhere anywhere
ACCEPT all -- anywhere anywhere
ACCEPT all -- anywhere anywhere
ACCEPT all -- anywhere anywhere ctstate RELATED,ESTABLISHED
DOCKER all -- anywhere anywhere
ACCEPT all -- anywhere anywhere
ACCEPT all -- anywhere anywhere
ACCEPT all -- anywhere anywhere ctstate RELATED,ESTABLISHED
DOCKER all -- anywhere anywhere
ACCEPT all -- anywhere anywhere
ACCEPT all -- anywhere anywhere
LIBVIRT_FWX all -- anywhere anywhere
LIBVIRT_FWI all -- anywhere anywhere
LIBVIRT_FWO all -- anywhere anywhere
Chain OUTPUT (policy ACCEPT)
target prot opt source destination
LIBVIRT_OUT all -- anywhere anywhere
Chain DOCKER (6 references)
target prot opt source destination
ACCEPT tcp -- anywhere 172.21.0.2 tcp dpt:https
ACCEPT tcp -- anywhere 172.21.0.2 tcp dpt:http
Chain DOCKER-ISOLATION-STAGE-1 (1 references)
target prot opt source destination
DOCKER-ISOLATION-STAGE-2 all -- anywhere anywhere
DOCKER-ISOLATION-STAGE-2 all -- anywhere anywhere
DOCKER-ISOLATION-STAGE-2 all -- anywhere anywhere
DOCKER-ISOLATION-STAGE-2 all -- anywhere anywhere
DOCKER-ISOLATION-STAGE-2 all -- anywhere anywhere
DOCKER-ISOLATION-STAGE-2 all -- anywhere anywhere
RETURN all -- anywhere anywhere
Chain DOCKER-ISOLATION-STAGE-2 (6 references)
target prot opt source destination
DROP all -- anywhere anywhere
DROP all -- anywhere anywhere
DROP all -- anywhere anywhere
DROP all -- anywhere anywhere
DROP all -- anywhere anywhere
DROP all -- anywhere anywhere
RETURN all -- anywhere anywhere
Chain DOCKER-USER (1 references)
target prot opt source destination
RETURN all -- anywhere anywhere
Chain LIBVIRT_FWI (1 references)
target prot opt source destination
ACCEPT all -- anywhere 192.168.100.0/24 ctstate RELATED,ESTABLISHED
REJECT all -- anywhere anywhere reject-with icmp-port-unreachable
Chain LIBVIRT_FWO (1 references)
target prot opt source destination
ACCEPT all -- 192.168.100.0/24 anywhere
REJECT all -- anywhere anywhere reject-with icmp-port-unreachable
Chain LIBVIRT_FWX (1 references)
target prot opt source destination
ACCEPT all -- anywhere anywhere
Chain LIBVIRT_INP (1 references)
target prot opt source destination
ACCEPT udp -- anywhere anywhere udp dpt:domain
ACCEPT tcp -- anywhere anywhere tcp dpt:domain
ACCEPT udp -- anywhere anywhere udp dpt:bootps
ACCEPT tcp -- anywhere anywhere tcp dpt:67
Chain LIBVIRT_OUT (1 references)
target prot opt source destination
ACCEPT udp -- anywhere anywhere udp dpt:domain
ACCEPT tcp -- anywhere anywhere tcp dpt:domain
ACCEPT udp -- anywhere anywhere udp dpt:bootpc
ACCEPT tcp -- anywhere anywhere tcp dpt:68
|
Firewalld
Specifically used in Fedora, firewalld
serves as a user-friendly
frontend for iptables
, simplifying firewall management.
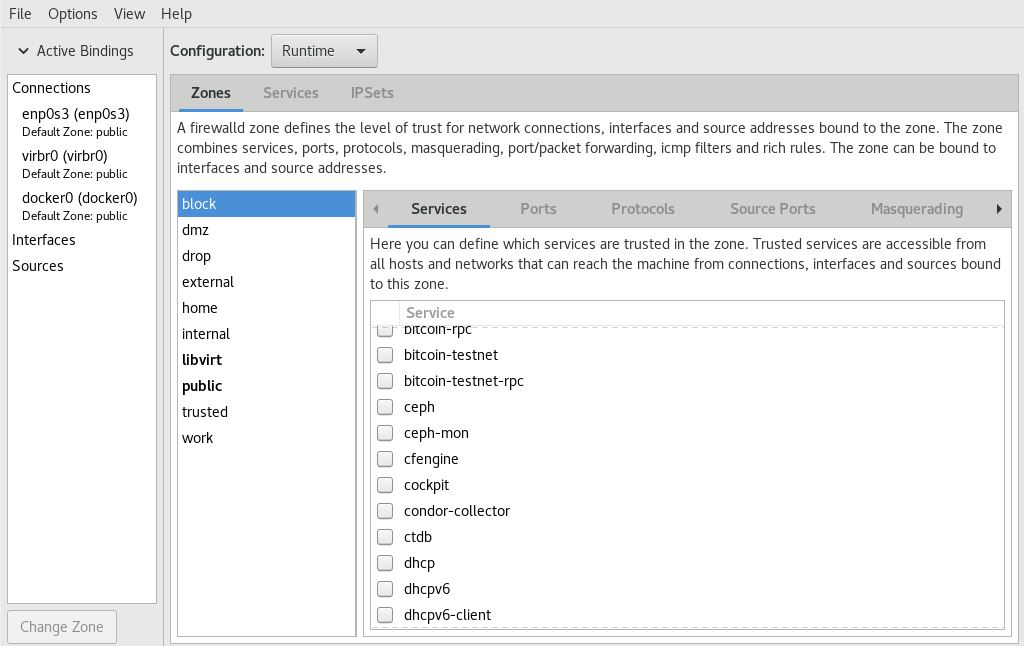